Okay, so today I decided to play around with making a simple Snake game. I’ve always been a bit fascinated by those retro games, and I figured, why not try to build one myself? I’m not a pro developer or anything, just someone who likes to tinker.
Getting Started
First things first, I needed to choose a tool. I didn’t want anything too complicated, I just wanted to get it implemented. So, after I made some research I decided to go with Python and the `pygame` library. I’ve dabbled with Python before, and `pygame` seemed pretty straightforward for handling graphics and input.
I started by installing `pygame`. It was as simple as opening up my terminal and typing:
pip install pygame
The Basic Structure
Then I started a new Python file. I initialized `pygame`, set up the basic game window, and created a simple game loop. You know, the usual stuff – a `while` loop that keeps running until you tell it to stop. Inside, I added some code to handle events (like key presses) and update the game elements.
Drawing the Snake and Food
Next up, I had to figure out how to draw the snake. I decided to represent the snake as a list of segments, each segment being a simple rectangle. I created a function to draw these rectangles on the screen. For the food, I just drew another rectangle at a random position.
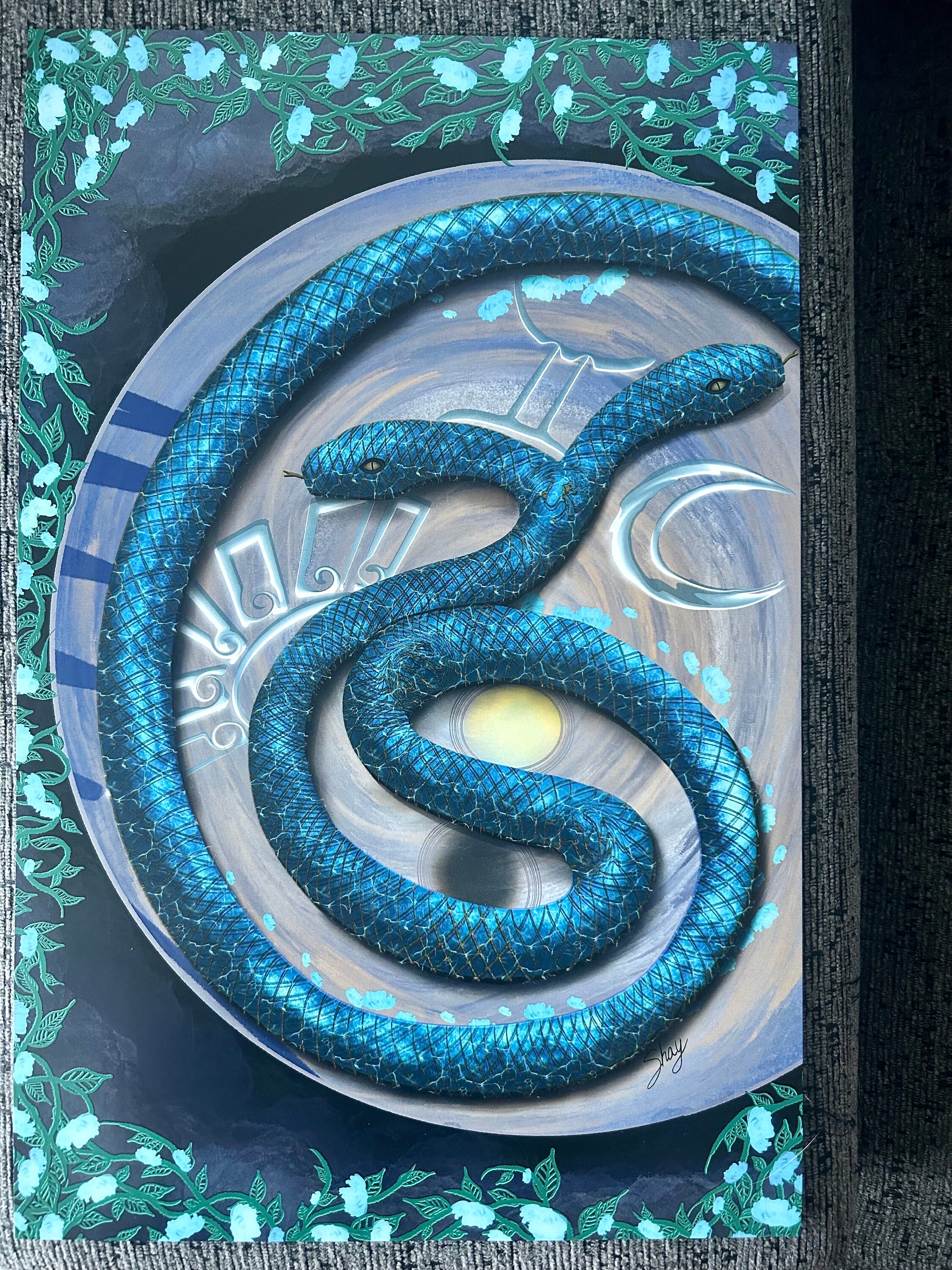
Making the Snake Move
This was the tricky part, I spent a lot of time to implemented it. I needed to make the snake move continuously in one direction. I used a simple approach: every few frames, I added a new segment to the head of the snake in the current direction, and I removed the tail segment. This created the illusion of movement. I added some code to change the direction based on arrow key presses.
Collision Detection
Of course, a Snake game needs collision detection! I added some checks to see if the snake’s head collided with the food. If it did, I increased the score and moved the food to a new random location. Also the new food will increase snake’s length. I also added checks to see if the snake collided with the edges of the screen or with itself. If either of those happened, it was game over.
Displaying the Score
I needed a way to display the score, right? I used `pygame`’s font functions to render text on the screen, showing the current score. Nothing fancy, just a simple number in the corner.
The Finished Product (Well, Sort Of)
After a few hours of coding and debugging, I had a working Snake game! It wasn’t perfect – the graphics were basic, and there were probably some bugs lurking – but it worked! I could control the snake, eat the food, and the game would end if I crashed. I’m pretty happy how it came out.
It was a fun little project, and I learned a few things along the way. Maybe I’ll add some more features later, like different levels or power-ups. But for now, I’m just happy I managed to build a working game from scratch!